Conflicts are one of the center topic about abetooDB. Conflicts are triggered whenever the revision chain is broken and it could happend if more than one writer have created a new revision over the same document,while they are offline, and then they try to syncronize its revision with each other. It could also happend, if within the same database we have two version of the same document.
Tip
A Conflict is triggered whenever we try to save a new revision of a document, breaking its revision chain within the database.
But first let’s explain how the document revisions chain works in abetooDB.
Whenever a new revision of a document is stored in the database a new revision identifier is created with it. So after several updates have taken place, a revision chain, like the following one, is forming.
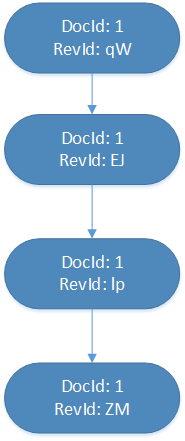
In the example above every revision has a two letters identifier, generated randomly. This is just an example for illustration purpose, in reality the abetooDB document revision identifier and its revision identifiers are composed by a larger signature to give a guarantee that there will be no collisions. Every document identifier and revision identifier is unique along, not only the local database, but all the distributed databases.
We can access to any previous revision using the HistoryRevisions document property. Revisions are sorted by order of arrival, most recent first.
Tip
The property HistoryRevisions of a document contains the revisions chain. Revisions are sorted by order of arrival, most recent first.
How to trigger a conflict?
Let’s trigger a conflict, to do that we have to try to insert a new revision in the document but with an old version of it. Let’s do it against the local database, so first insert a document in the database,
// Instantiate a new database
AbtooDB dbA = AbtooDB.getDB("testconflictsdb");
// Define a conflict resolution function
dbA.OnConflict += ((sender, e) =>
{
e.winnerrev = null; // pick no one revision as a winner
});
// Insert a document
AbtooDocument car = AbtooDocument.createDocument(dbA, "cars");
Dictionary<string, object> properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 120);
properties.Add("airbag", true);
car.update(properties);
So now we have a document with a single revision, the current one,
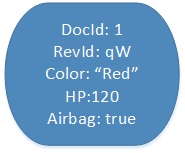
Now retrieve two references of the document we’ve created above,
// Retrieve two references of the created document
AbtooDocument car1 = AbtooDocument.getDocById(dbA, car.DocId);
AbtooDocument car2 = AbtooDocument.getDocById(dbA, car.DocId);
Update car1 with a new set of properties generating a new revision,
// Update car1 reference with a new revision
properties = new Dictionary<string, object>();
properties.Add("color", "Blue");
properties.Add("HP", 130);
properties.Add("airbag", false);
car1.update(properties);
Updating the document revision chain, what we have is,
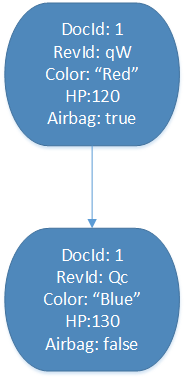
Without retrieving again car2 from the database, remember that car2 is referencing to an older revision of the document, let’s update car2 with a new set of properties, this operation will trigger a conflict,
// Update car2 reference with a new revision,
// but car2 is an "old" version => trigger a conflict.
properties = new Dictionary<string, object>();
properties.Add("color", "Orange");
properties.Add("HP", 140);
properties.Add("airbag", true);
car2.update(properties);
The document revisions chain looks like something like this,
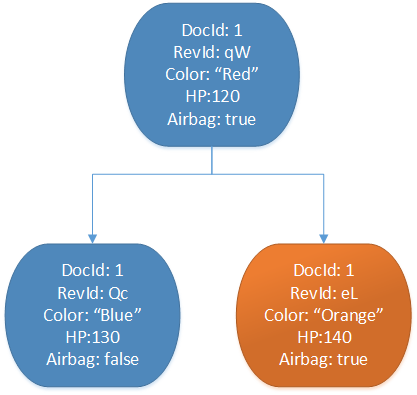
We can know if a document has any conflicts with the method hasConflict,
Console.WriteLine(car2.hasConflict()); // true
Console.WriteLine(car2.getString("color")); // Blue
Notice how when we retrieve the current value of the color property we get the Blue value, this is due to the fact that this was the last revision inserted within the database with no conflicts. So the CurrentRevision of the document is referencing to the revision with the RevId: Qc.
But what about if we want to access to the conflicting revision(s) of the document, we can do so by accessing the the ConflictRevisions property of the document,
AbtooRev conflictrevision = car2.ConflictRevisions[0];
Console.WriteLine(conflictrevision.getString("color")); // Orange
So the first item in the collection of the conflicting revisions correspond to the revision with the RevId: eL, with an Orange value for the color property.
The example above was exposed just for illustration purpose, usually conflicts are triggered by sync processes running in the background.
How to solve a conflict?
Notice how at the very beggining of the definition of the database in the example above, we’ve introduced the following lines of code,
AbtooDB dbA = AbtooDB.getDB("testconflictsdb");
// Define a conflict resolution function
dbA.OnConflict += ((sender, e) =>
{
e.winnerrev = null;
});
As an advance, the meaning of this lines is to let the user solve the conflict later.
abetooDB let’s we solve the conflicts in three different ways. This is up to the developer to define the way that fits better for his application.
But there is a very important question, due to the fact that abetooDB is a distributed database, whenever we define a conflict resolution function, it has to be consistent along the whole databases. All of them have to do exactly the same withouth need to interchange any message with each other. The conflict resolution function has to generate exactly the same result for a set of input revisions along the whole databases.
Tip
Due to the fact that abetooDB is a distributed database, whenever we define a conflict resolution function, it has to be consistent along the whole databases. All of them have to do exactly the same withouth need to interchange any message with each other!!. The conflict resolution function has to generate exactly the same result for a set of input revisions along the whole databases.
Automatic conflict resolution
If we don’t define any conflict resolution function, like it was done before, abetooDB will solve any conflict for us automatically. And It will do it picking the revision with the larger timestamp as the winner one. So if we replicate the example above with no conflict resolution function, the conflict will appear, but it will be automatically solved by abetooDB.
// Instantiate a new database
// No conflict resolution function
AbtooDB dbA = AbtooDB.getDB("testconflictsdb");
// Insert a document
AbtooDocument car = AbtooDocument.createDocument(dbA, "cars");
Dictionary<string, object> properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 120);
properties.Add("airbag", true);
car.update(properties);
// Retrieve two references of the created document
AbtooDocument car1 = AbtooDocument.getDocById(dbA, car.DocId);
AbtooDocument car2 = AbtooDocument.getDocById(dbA, car.DocId);
// Update car1 reference with a new revision
properties = new Dictionary<string, object>();
properties.Add("color", "Blue");
properties.Add("HP", 130);
properties.Add("airbag", false);
car1.update(properties);
// Update car2 reference with a new revision,
// but car2 is an "old" version => trigger a conflict.
// but is solved by abetooDB
properties = new Dictionary<string, object>();
properties.Add("color", "Orange");
properties.Add("HP", 140);
properties.Add("airbag", true);
car2.update(properties);
Console.WriteLine(car2.hasConflict()); // false
Console.WriteLine(car2.getString("color")); // Orange
Notice how now the code is exactly the same but without any conflict resolution function. Conflict is triggered and solved internally, the last inserted revision is picked as the winner one.
This functionality could be very usefull if we are working with time based revisions, and what we want is to solve any conflict getting the last revision generated as the winner one. Notice that abetooDB pick the revision with the larger timestamp as the winner one, not the last inserted. This could be very important in a distributed enviroment.
Picking the winner revision manually, whenever a conflict occur
This is a very useful feature that lets the developer take a full control about the question of what revision have to be taken as the winner one, for every single situation.
Maybe if we have different types of documents like cars, colors, etc, like it was exposed in some previous examples, we could be interested into apply some conflict resolution algorithm over one type, different from the others.
Let’s rewrite the example above but this time picking the current revision as the winner one,
// Instantiate a new database
// Define a conflict resolution function
// that picks the current revision as the winner one
AbtooDB dbA = AbtooDB.getDB("testconflictsdb");
dbA.OnConflict += ((sender, revisionslist) =>
{
AbtooRev currentrev = revisionslist.confictsrevs[0];
revisionslist.winnerrev = currentrev;
});
// Insert a document
AbtooDocument car = AbtooDocument.createDocument(dbA, "cars");
Dictionary<string, object> properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 120);
properties.Add("airbag", true);
car.update(properties);
// Retrieve two references of the created document
AbtooDocument car1 = AbtooDocument.getDocById(dbA, car.DocId);
AbtooDocument car2 = AbtooDocument.getDocById(dbA, car.DocId);
// Update car1 reference with a new revision
properties = new Dictionary<string, object>();
properties.Add("color", "Blue");
properties.Add("HP", 130);
properties.Add("airbag", false);
car1.update(properties);
// Update car2 reference with a new revision,
// but car2 is an "old" version => trigger a conflict.
// but is solved by the current resolution function
properties = new Dictionary<string, object>();
properties.Add("color", "Orange");
properties.Add("HP", 140);
properties.Add("airbag", true);
car2.update(properties);
Console.WriteLine(car2.hasConflict()); // false
Console.WriteLine(car2.getString("color")); // Blue
Notice how now despite of we’ve inserted the Orange revision triggering a conflict, the conflict resolution function picks the first revision as the winner one, which is the current revision, the last valid one.
Picking the winner revision manually, but with the participation of the end user
What about if at the moment the conflict take place we cannot pick any revision as a winner because we cannot define a fixed resolution algorithm for every single situation. This is a very interesting feature, mostly used whenever we need the participation of the final user in order to solve the conflict.
Imagine that we have two different revisions of the same document in conflict, and the conflict is related to some kind of information understandable only by the end user. Following the previous examples, let’s create a new conflict,
// Instantiate a new database
// Define a conflict resolution function
AbtooDB dbA = AbtooDB.getDB("testconflictsdb");
dbA.OnConflict += ((sender, e) =>
{
if (e.doc.DocType == "cars")
{
e.winnerrev = null; // let the user decide
}
else
{
e.winnerrev = e.confictsrevs[0];
}
});
// Insert a document
AbtooDocument car = AbtooDocument.createDocument(dbA, "cars");
Dictionary<string, object> properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 120);
properties.Add("airbag", true);
car.update(properties);
// Retrieve two references of the created document
AbtooDocument car1 = AbtooDocument.getDocById(dbA, car.DocId);
AbtooDocument car2 = AbtooDocument.getDocById(dbA, car.DocId);
// Update car1 reference with a new revision
properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 130);
properties.Add("airbag", false);
car1.update(properties);
// Update car2 reference with a new revision,
// but car2 is an "old" version => trigger a conflict.
properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 140);
properties.Add("airbag", false);
car2.update(properties);
// Check, conflict is triggered
Console.WriteLine(car2.hasConflict()); // true
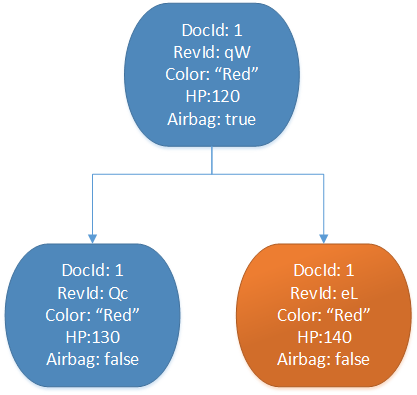
Now we have a document with two different revisions in conflict, one with HP 130 and other with HP 140. We cannot pick any of these revisions as a winner one automatically, because we don’t have a meaningful way to know which one is the right one. We need the participation of the end user, maybe showing these conflict revisions in a GUI and letting the user pick one of them for us.
// let the user decide which revision is the right one
// ...
// Show revisions in a GUI and let the user pick one
// ...
// ...
// user gets the current revision as the right one
AbtooRev winrev = car2.CurrentRevision;
Once we have a valid revision and we want to persist it into the database to solve the conflict, we can call the method solveConflict, with just a single parameter, the revision we want to be the current one.
// Solve conflict
car2.solveConflict(winrev);
And now if we test again to know if there are any conflict within the document, we get,
// Check, conflict is triggered
Console.WriteLine(car2.hasConflict()); // false
Console.WriteLine(car2.getInt("HP")); // 130
So the conflict is gone.
But what about if what we want is not to pick an existing revision, but to create a new one merging properties of two or more revisions.
Tip
If what we want is not to pick an existing revision, but to create a new one merging properties of two or more revisions, don’t modify the properties of an existing revision selecting it as the winner one!!. This could lead to an unpredictable database behaviour. abetooDB has a mechanism to this situation.
Following the example above but unlike to select a revision between the existing ones. Now let’s create another new revision with a new set of properties,
// let the user decide which revision is the right one
// ...
// Show revisions in a GUI
// ...
// ...
// user sets the arithmetic average as the right HP value
properties = new Dictionary<string, object>();
properties.Add("color", "Red");
properties.Add("HP", 135);
properties.Add("airbag", false);
AbtooRev winrev = dbA.createRevision(properties);
// Solve conflict
car2.solveConflict(winrev);
// Check, conflict is triggered
Console.WriteLine(car2.hasConflict()); // false
Console.WriteLine(car2.getInt("HP")); // 135
How to know if there are any document with conflicts?
We can retrieve the collection of documents with conflitc(s) at any given time by calling the getConflictDocs method this way,
List<AbtooDocument> conflictdocs = dbA.getConflitcDocs();
Then we can iterate over the collection solving the conflicts as it was described before.